mirror of
https://github.com/bytecodealliance/wasm-micro-runtime.git
synced 2025-02-11 09:25:20 +00:00
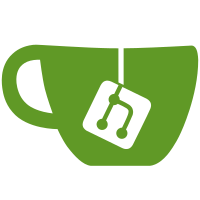
- Add new API wasm_runtime_load_ex() in wasm_export.h and wasm_module_new_ex in wasm_c_api.h - Put aot_create_perf_map() into a separated file aot_perf_map.c - In perf.map, function names include user specified module name - Enhance the script to help flamegraph generations
39 lines
649 B
C
39 lines
649 B
C
#include <stdio.h>
|
|
|
|
// Ackermann function
|
|
unsigned long
|
|
ackermann(unsigned long m, unsigned long n)
|
|
{
|
|
if (m == 0) {
|
|
return n + 1;
|
|
}
|
|
else if (n == 0) {
|
|
return ackermann(m - 1, 1);
|
|
}
|
|
else {
|
|
return ackermann(m - 1, ackermann(m, n - 1));
|
|
}
|
|
}
|
|
|
|
__attribute__((export_name("run"))) int
|
|
run(int m, int n)
|
|
{
|
|
int result = ackermann(m, n);
|
|
printf("ackermann(%d, %d)=%d\n", m, n, result);
|
|
return result;
|
|
}
|
|
|
|
int
|
|
main()
|
|
{
|
|
unsigned long m, n, result;
|
|
|
|
// Example usage:
|
|
m = 3;
|
|
n = 2;
|
|
result = ackermann(m, n);
|
|
printf("Ackermann(%lu, %lu) = %lu\n", m, n, result);
|
|
|
|
return 0;
|
|
}
|