mirror of
https://github.com/bytecodealliance/wasm-micro-runtime.git
synced 2025-05-09 13:16:26 +00:00
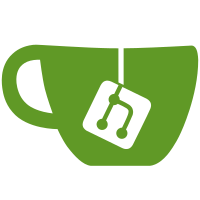
Tests were failing because the right permissions were not provided to iwasm. Also, test failures didn't trigger build failure due to typo - also fixed in this change. In addition to that, this PR fixes a few issues with the test itself: * the `server_init_complete` was not reset early enough causing the client to occasionally assume the server started even though it didn't yet * set `SO_REUSEADDR` on the server socket so the port can be reused shortly after closing the previous socket * defined receive-send-receive sequence from server to make sure server is alive at the time of sending message
69 lines
1.4 KiB
C
69 lines
1.4 KiB
C
/*
|
|
* Copyright (C) 2023 Amazon.com Inc. or its affiliates. All rights reserved.
|
|
* SPDX-License-Identifier: Apache-2.0 WITH LLVM-exception
|
|
*/
|
|
|
|
#include <assert.h>
|
|
#include <string.h>
|
|
#include <stdio.h>
|
|
#include <pthread.h>
|
|
#ifdef __wasi__
|
|
#include <wasi/api.h>
|
|
#include <sys/socket.h>
|
|
#include <netinet/in.h>
|
|
#include <wasi_socket_ext.h>
|
|
#else
|
|
#include <netdb.h>
|
|
#endif
|
|
|
|
void
|
|
test_nslookup(int af)
|
|
{
|
|
struct addrinfo *res;
|
|
int count = 0;
|
|
struct addrinfo hints;
|
|
char *url = "google-public-dns-a.google.com";
|
|
|
|
memset(&hints, 0, sizeof(hints));
|
|
hints.ai_family = af;
|
|
hints.ai_socktype = SOCK_STREAM;
|
|
int ret = getaddrinfo(url, 0, &hints, &res);
|
|
assert(ret == 0);
|
|
struct addrinfo *address = res;
|
|
while (address) {
|
|
assert(address->ai_family == af);
|
|
assert(address->ai_socktype == SOCK_STREAM);
|
|
count++;
|
|
address = address->ai_next;
|
|
}
|
|
|
|
assert(count > 0);
|
|
freeaddrinfo(res);
|
|
}
|
|
|
|
void *
|
|
test_nslookup_mt(void *params)
|
|
{
|
|
int *af = (int *)params;
|
|
test_nslookup(*af);
|
|
return NULL;
|
|
}
|
|
|
|
int
|
|
main()
|
|
{
|
|
int afs[] = { AF_INET, AF_INET6 };
|
|
|
|
for (int i = 0; i < sizeof(afs) / sizeof(afs[0]); i++) {
|
|
pthread_t th;
|
|
|
|
printf("Testing %d in main thread...\n", afs[i]);
|
|
test_nslookup(afs[i]);
|
|
printf("Testing %d in a new thread...\n", afs[i]);
|
|
pthread_create(&th, NULL, test_nslookup_mt, &afs[i]);
|
|
pthread_join(th, NULL);
|
|
}
|
|
|
|
return 0;
|
|
}
|