mirror of
https://github.com/bytecodealliance/wasm-micro-runtime.git
synced 2024-11-26 15:32:05 +00:00
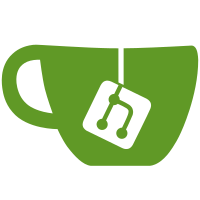
# Change the data type representing linear memory address from u32 to u64 ## APIs signature changes - (Export)wasm_runtime_module_malloc - wasm_module_malloc - wasm_module_malloc_internal - aot_module_malloc - aot_module_malloc_internal - wasm_runtime_module_realloc - wasm_module_realloc - wasm_module_realloc_internal - aot_module_realloc - aot_module_realloc_internal - (Export)wasm_runtime_module_free - wasm_module_free - wasm_module_free_internal - aot_module_malloc - aot_module_free_internal - (Export)wasm_runtime_module_dup_data - wasm_module_dup_data - aot_module_dup_data - (Export)wasm_runtime_validate_app_addr - (Export)wasm_runtime_validate_app_str_addr - (Export)wasm_runtime_validate_native_addr - (Export)wasm_runtime_addr_app_to_native - (Export)wasm_runtime_addr_native_to_app - (Export)wasm_runtime_get_app_addr_range - aot_set_aux_stack - aot_get_aux_stack - wasm_set_aux_stack - wasm_get_aux_stack - aot_check_app_addr_and_convert, wasm_check_app_addr_and_convert and jit_check_app_addr_and_convert - wasm_exec_env_set_aux_stack - wasm_exec_env_get_aux_stack - wasm_cluster_create_thread - wasm_cluster_allocate_aux_stack - wasm_cluster_free_aux_stack ## Data structure changes - WASMModule and AOTModule - field aux_data_end, aux_heap_base and aux_stack_bottom - WASMExecEnv - field aux_stack_boundary and aux_stack_bottom - AOTCompData - field aux_data_end, aux_heap_base and aux_stack_bottom - WASMMemoryInstance(AOTMemoryInstance) - field memory_data_size and change __padding to is_memory64 - WASMModuleInstMemConsumption - field total_size and memories_size - WASMDebugExecutionMemory - field start_offset and current_pos - WASMCluster - field stack_tops ## Components that are affected by the APIs and data structure changes - libc-builtin - libc-emcc - libc-uvwasi - libc-wasi - Python and Go Language Embedding - Interpreter Debug engine - Multi-thread: lib-pthread, wasi-threads and thread manager
75 lines
2.0 KiB
C
75 lines
2.0 KiB
C
/*
|
|
* Copyright (C) 2019 Intel Corporation. All rights reserved.
|
|
* SPDX-License-Identifier: Apache-2.0 WITH LLVM-exception
|
|
*/
|
|
|
|
/*
|
|
* This example basically does the same thing as test_hello.c,
|
|
* using wasm_export.h API.
|
|
*/
|
|
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
|
|
#include "wasm_export.h"
|
|
|
|
static int
|
|
test_hello2_wrapper(wasm_exec_env_t exec_env, uint32_t nameaddr,
|
|
uint32_t resultaddr, uint32_t resultlen)
|
|
{
|
|
/*
|
|
* Perform wasm_runtime_malloc to check if the runtime has been
|
|
* initialized as expected.
|
|
* This would fail with "memory hasn't been initialize" error
|
|
* unless we are not sharing a runtime with the loader app. (iwasm)
|
|
*/
|
|
void *p = wasm_runtime_malloc(1);
|
|
if (p == NULL) {
|
|
return -1;
|
|
}
|
|
wasm_runtime_free(p);
|
|
|
|
wasm_module_inst_t inst = wasm_runtime_get_module_inst(exec_env);
|
|
if (!wasm_runtime_validate_app_str_addr(inst, (uint64_t)nameaddr)
|
|
|| !wasm_runtime_validate_app_addr(inst, (uint64_t)resultaddr,
|
|
(uint64_t)resultlen)) {
|
|
return -1;
|
|
}
|
|
const char *name =
|
|
wasm_runtime_addr_app_to_native(inst, (uint64_t)nameaddr);
|
|
char *result = wasm_runtime_addr_app_to_native(inst, (uint64_t)resultaddr);
|
|
return snprintf(result, resultlen,
|
|
"Hello, %s. This is %s! Your wasm_module_inst_t is %p.\n",
|
|
name, __func__, inst);
|
|
}
|
|
|
|
/* clang-format off */
|
|
#define REG_NATIVE_FUNC(func_name, signature) \
|
|
{ #func_name, func_name##_wrapper, signature, NULL }
|
|
|
|
static NativeSymbol native_symbols[] = {
|
|
REG_NATIVE_FUNC(test_hello2, "(iii)i")
|
|
};
|
|
/* clang-format on */
|
|
|
|
uint32_t
|
|
get_native_lib(char **p_module_name, NativeSymbol **p_native_symbols)
|
|
{
|
|
*p_module_name = "env";
|
|
*p_native_symbols = native_symbols;
|
|
return sizeof(native_symbols) / sizeof(NativeSymbol);
|
|
}
|
|
|
|
int
|
|
init_native_lib()
|
|
{
|
|
printf("%s in test_hello2.c called\n", __func__);
|
|
return 0;
|
|
}
|
|
|
|
void
|
|
deinit_native_lib()
|
|
{
|
|
printf("%s in test_hello2.c called\n", __func__);
|
|
}
|