mirror of
https://github.com/bytecodealliance/wasm-micro-runtime.git
synced 2024-10-16 13:02:32 +00:00
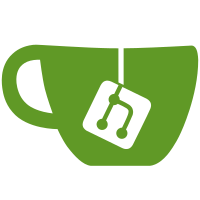
Currently, `data.drop` instruction is implemented by directly modifying the underlying module. It breaks use cases where you have multiple instances sharing a single loaded module. `elem.drop` has the same problem too. This PR fixes the issue by keeping track of which data/elem segments have been dropped by using bitmaps for each module instances separately, and add a sample to demonstrate the issue and make the CI run it. Also add a missing check of dropped elements to the fast-jit `table.init`. Fixes: https://github.com/bytecodealliance/wasm-micro-runtime/issues/2735 Fixes: https://github.com/bytecodealliance/wasm-micro-runtime/issues/2772
28 lines
758 B
C
28 lines
758 B
C
/*
|
|
* Copyright (C) 2021 Intel Corporation. All rights reserved.
|
|
* SPDX-License-Identifier: Apache-2.0 WITH LLVM-exception
|
|
*/
|
|
|
|
#include "bh_bitmap.h"
|
|
|
|
bh_bitmap *
|
|
bh_bitmap_new(uintptr_t begin_index, unsigned bitnum)
|
|
{
|
|
bh_bitmap *bitmap;
|
|
uint32 bitmap_size = (bitnum + 7) / 8;
|
|
uint32 total_size = offsetof(bh_bitmap, map) + bitmap_size;
|
|
|
|
if (bitnum > UINT32_MAX - 7 || total_size < offsetof(bh_bitmap, map)
|
|
|| (total_size - offsetof(bh_bitmap, map)) != bitmap_size) {
|
|
return NULL; /* integer overflow */
|
|
}
|
|
|
|
if ((bitmap = BH_MALLOC(total_size)) != NULL) {
|
|
memset(bitmap, 0, total_size);
|
|
bitmap->begin_index = begin_index;
|
|
bitmap->end_index = begin_index + bitnum;
|
|
}
|
|
|
|
return bitmap;
|
|
}
|