mirror of
https://github.com/bytecodealliance/wasm-micro-runtime.git
synced 2025-06-18 02:59:21 +00:00
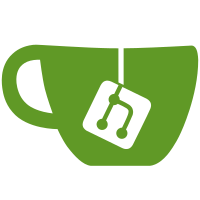
it is allowed that all imported functions and globals can be linked by multi-module feature automatically or by wasm-c-api manually
48 lines
1.1 KiB
C
48 lines
1.1 KiB
C
/*
|
|
* Copyright (C) 2019 Intel Corporation. All rights reserved.
|
|
* SPDX-License-Identifier: Apache-2.0 WITH LLVM-exception
|
|
*/
|
|
|
|
#include "bh_read_file.h"
|
|
#include "wasm_export.h"
|
|
|
|
static char *
|
|
build_module_path(const char *module_name)
|
|
{
|
|
const char *module_search_path = ".";
|
|
const char *format = "%s/%s.wasm";
|
|
int sz = strlen(module_search_path) + strlen("/") + strlen(module_name)
|
|
+ strlen(".wasm") + 1;
|
|
char *wasm_file_name = BH_MALLOC(sz);
|
|
if (!wasm_file_name) {
|
|
return NULL;
|
|
}
|
|
|
|
snprintf(wasm_file_name, sz, format, module_search_path, module_name);
|
|
return wasm_file_name;
|
|
}
|
|
|
|
bool
|
|
reader(const char *module_name, uint8 **p_buffer, uint32 *p_size)
|
|
{
|
|
char *wasm_file_path = build_module_path(module_name);
|
|
if (!wasm_file_path) {
|
|
return false;
|
|
}
|
|
|
|
*p_buffer = (uint8_t *)bh_read_file_to_buffer(wasm_file_path, p_size);
|
|
BH_FREE(wasm_file_path);
|
|
return *p_buffer != NULL;
|
|
}
|
|
|
|
void
|
|
destroyer(uint8 *buffer, uint32 size)
|
|
{
|
|
if (!buffer) {
|
|
return;
|
|
}
|
|
|
|
BH_FREE(buffer);
|
|
buffer = NULL;
|
|
}
|