mirror of
https://github.com/bytecodealliance/wasm-micro-runtime.git
synced 2025-07-10 06:23:45 +00:00
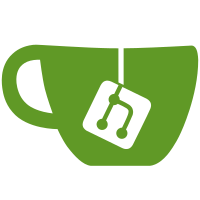
Use LLVM new pass manager for wamrc to replace the legacy pass manger, so as to gain better performance and reduce the compilation time. Reference links: - https://llvm.org/docs/NewPassManager.html - https://blog.llvm.org/posts/2021-03-26-the-new-pass-manager And add an option to use the legacy pm mode when building wamrc: cmake .. -DWAMR_BUILD_LLVM_LEGACY_PM=1 For JIT mode, keep it unchanged as it only runs several function passes and using new pass manager will increase the compilation time. And refactor the codes of applying LLVM passes.
97 lines
2.0 KiB
C
97 lines
2.0 KiB
C
/*
|
|
* Copyright (C) 2019 Intel Corporation. All rights reserved.
|
|
* SPDX-License-Identifier: Apache-2.0 WITH LLVM-exception
|
|
*/
|
|
|
|
#ifndef _AOT_EXPORT_H
|
|
#define _AOT_EXPORT_H
|
|
|
|
#include <stdint.h>
|
|
#include <stdbool.h>
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
struct AOTCompData;
|
|
typedef struct AOTCompData *aot_comp_data_t;
|
|
|
|
struct AOTCompContext;
|
|
typedef struct AOTCompContext *aot_comp_context_t;
|
|
|
|
aot_comp_data_t
|
|
aot_create_comp_data(void *wasm_module);
|
|
|
|
void
|
|
aot_destroy_comp_data(aot_comp_data_t comp_data);
|
|
|
|
#if WASM_ENABLE_DEBUG_AOT != 0
|
|
typedef void *dwar_extractor_handle_t;
|
|
dwar_extractor_handle_t
|
|
create_dwarf_extractor(aot_comp_data_t comp_data, char *file_name);
|
|
#endif
|
|
|
|
enum {
|
|
AOT_FORMAT_FILE,
|
|
AOT_OBJECT_FILE,
|
|
AOT_LLVMIR_UNOPT_FILE,
|
|
AOT_LLVMIR_OPT_FILE,
|
|
};
|
|
|
|
typedef struct AOTCompOption {
|
|
bool is_jit_mode;
|
|
bool is_indirect_mode;
|
|
char *target_arch;
|
|
char *target_abi;
|
|
char *target_cpu;
|
|
char *cpu_features;
|
|
bool is_sgx_platform;
|
|
bool enable_bulk_memory;
|
|
bool enable_thread_mgr;
|
|
bool enable_tail_call;
|
|
bool enable_simd;
|
|
bool enable_ref_types;
|
|
bool enable_aux_stack_check;
|
|
bool enable_aux_stack_frame;
|
|
bool disable_llvm_intrinsics;
|
|
bool disable_llvm_lto;
|
|
uint32_t opt_level;
|
|
uint32_t size_level;
|
|
uint32_t output_format;
|
|
uint32_t bounds_checks;
|
|
} AOTCompOption, *aot_comp_option_t;
|
|
|
|
aot_comp_context_t
|
|
aot_create_comp_context(aot_comp_data_t comp_data, aot_comp_option_t option);
|
|
|
|
void
|
|
aot_destroy_comp_context(aot_comp_context_t comp_ctx);
|
|
|
|
bool
|
|
aot_compile_wasm(aot_comp_context_t comp_ctx);
|
|
|
|
bool
|
|
aot_emit_llvm_file(aot_comp_context_t comp_ctx, const char *file_name);
|
|
|
|
bool
|
|
aot_emit_object_file(aot_comp_context_t comp_ctx, const char *file_name);
|
|
|
|
bool
|
|
aot_emit_aot_file(aot_comp_context_t comp_ctx, aot_comp_data_t comp_data,
|
|
const char *file_name);
|
|
|
|
void
|
|
aot_destroy_aot_file(uint8_t *aot_file);
|
|
|
|
char *
|
|
aot_get_last_error();
|
|
|
|
uint32_t
|
|
aot_get_plt_table_size();
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif /* end of _AOT_EXPORT_H */
|