mirror of
https://github.com/bytecodealliance/wasm-micro-runtime.git
synced 2025-02-06 23:15:16 +00:00
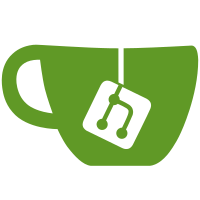
# Change the data type representing linear memory address from u32 to u64 ## APIs signature changes - (Export)wasm_runtime_module_malloc - wasm_module_malloc - wasm_module_malloc_internal - aot_module_malloc - aot_module_malloc_internal - wasm_runtime_module_realloc - wasm_module_realloc - wasm_module_realloc_internal - aot_module_realloc - aot_module_realloc_internal - (Export)wasm_runtime_module_free - wasm_module_free - wasm_module_free_internal - aot_module_malloc - aot_module_free_internal - (Export)wasm_runtime_module_dup_data - wasm_module_dup_data - aot_module_dup_data - (Export)wasm_runtime_validate_app_addr - (Export)wasm_runtime_validate_app_str_addr - (Export)wasm_runtime_validate_native_addr - (Export)wasm_runtime_addr_app_to_native - (Export)wasm_runtime_addr_native_to_app - (Export)wasm_runtime_get_app_addr_range - aot_set_aux_stack - aot_get_aux_stack - wasm_set_aux_stack - wasm_get_aux_stack - aot_check_app_addr_and_convert, wasm_check_app_addr_and_convert and jit_check_app_addr_and_convert - wasm_exec_env_set_aux_stack - wasm_exec_env_get_aux_stack - wasm_cluster_create_thread - wasm_cluster_allocate_aux_stack - wasm_cluster_free_aux_stack ## Data structure changes - WASMModule and AOTModule - field aux_data_end, aux_heap_base and aux_stack_bottom - WASMExecEnv - field aux_stack_boundary and aux_stack_bottom - AOTCompData - field aux_data_end, aux_heap_base and aux_stack_bottom - WASMMemoryInstance(AOTMemoryInstance) - field memory_data_size and change __padding to is_memory64 - WASMModuleInstMemConsumption - field total_size and memories_size - WASMDebugExecutionMemory - field start_offset and current_pos - WASMCluster - field stack_tops ## Components that are affected by the APIs and data structure changes - libc-builtin - libc-emcc - libc-uvwasi - libc-wasi - Python and Go Language Embedding - Interpreter Debug engine - Multi-thread: lib-pthread, wasi-threads and thread manager
254 lines
7.0 KiB
C
254 lines
7.0 KiB
C
/*
|
|
* Copyright (C) 2021 Ant Group. All rights reserved.
|
|
* SPDX-License-Identifier: Apache-2.0 WITH LLVM-exception
|
|
*/
|
|
|
|
#ifndef _DEBUG_ENGINE_H
|
|
#define _DEBUG_ENGINE_H
|
|
|
|
#include "bh_list.h"
|
|
#include "gdbserver.h"
|
|
#include "thread_manager.h"
|
|
|
|
typedef enum WASMDebugControlThreadStatus {
|
|
RUNNING,
|
|
DETACHED,
|
|
STOPPED,
|
|
} WASMDebugControlThreadStatus;
|
|
|
|
struct WASMDebugEngine;
|
|
struct WASMDebugInstance;
|
|
|
|
typedef struct WASMDebugControlThread {
|
|
WASMGDBServer *server;
|
|
korp_tid tid;
|
|
korp_mutex wait_lock;
|
|
char ip_addr[128];
|
|
int port;
|
|
WASMDebugControlThreadStatus status;
|
|
struct WASMDebugEngine *debug_engine;
|
|
struct WASMDebugInstance *debug_instance;
|
|
} WASMDebugControlThread;
|
|
|
|
typedef struct WASMDebugBreakPoint {
|
|
struct WASMDebugBreakPoint *next;
|
|
uint64 addr;
|
|
uint64 orignal_data;
|
|
} WASMDebugBreakPoint;
|
|
|
|
typedef struct WASMDebugWatchPoint {
|
|
bh_list_link next;
|
|
uint64 addr;
|
|
uint64 length;
|
|
} WASMDebugWatchPoint;
|
|
|
|
typedef enum debug_state_t {
|
|
/* Debugger state conversion sequence:
|
|
* DBG_LAUNCHING ---> APP_STOPPED <---> APP_RUNNING
|
|
*/
|
|
DBG_LAUNCHING,
|
|
APP_RUNNING,
|
|
APP_STOPPED,
|
|
DBG_ERROR
|
|
} debug_state_t;
|
|
|
|
typedef struct WASMDebugExecutionMemory {
|
|
uint64 start_offset;
|
|
uint64 current_pos;
|
|
uint32 size;
|
|
} WASMDebugExecutionMemory;
|
|
|
|
struct WASMDebugInstance {
|
|
struct WASMDebugInstance *next;
|
|
WASMDebugControlThread *control_thread;
|
|
bh_list break_point_list;
|
|
bh_list watch_point_list_read;
|
|
bh_list watch_point_list_write;
|
|
WASMCluster *cluster;
|
|
uint32 id;
|
|
korp_tid current_tid;
|
|
korp_mutex wait_lock;
|
|
korp_cond wait_cond;
|
|
/* Last stopped thread, it should be set to NULL when sending
|
|
* out the thread stop reply */
|
|
WASMExecEnv *volatile stopped_thread;
|
|
/* Currently status of the debug instance, it will be set to
|
|
* RUNNING when receiving STEP/CONTINUE commands, and set to
|
|
* STOPPED when any thread stopped */
|
|
volatile debug_state_t current_state;
|
|
/* Execution memory info. During debugging, the debug client may request to
|
|
* malloc a memory space to evaluate user expressions. We preserve a buffer
|
|
* during creating debug instance, and use a simple bump pointer allocator
|
|
* to serve lldb's memory request */
|
|
WASMDebugExecutionMemory exec_mem_info;
|
|
};
|
|
|
|
typedef enum WASMDebugEventKind {
|
|
BREAK_POINT_ADD,
|
|
BREAK_POINT_REMOVE
|
|
} WASMDebugEventKind;
|
|
|
|
typedef struct WASMDebugEvent {
|
|
WASMDebugEventKind kind;
|
|
unsigned char metadata[0];
|
|
} WASMDebugEvent;
|
|
|
|
typedef struct WASMDebugMemoryInfo {
|
|
uint64 start;
|
|
uint64 size;
|
|
char name[128];
|
|
char permisson[4];
|
|
} WASMDebugMemoryInfo;
|
|
|
|
typedef enum WasmAddressType {
|
|
WasmMemory = 0x00,
|
|
WasmObj = 0x01,
|
|
WasmInvalid = 0x03
|
|
} WasmAddressType;
|
|
|
|
#define WASM_ADDR(type, id, offset) \
|
|
(((uint64)type << 62) | ((uint64)0 << 32) | ((uint64)offset << 0))
|
|
|
|
#define WASM_ADDR_TYPE(addr) (((addr)&0xC000000000000000) >> 62)
|
|
#define WASM_ADDR_OFFSET(addr) (((addr)&0x00000000FFFFFFFF))
|
|
|
|
#define INVALIED_ADDR (0xFFFFFFFFFFFFFFFF)
|
|
|
|
void
|
|
on_thread_stop_event(WASMDebugInstance *debug_inst, WASMExecEnv *exec_env);
|
|
|
|
void
|
|
on_thread_exit_event(WASMDebugInstance *debug_inst, WASMExecEnv *exec_env);
|
|
|
|
WASMDebugInstance *
|
|
wasm_debug_instance_create(WASMCluster *cluster, int32 port);
|
|
|
|
void
|
|
wasm_debug_instance_destroy(WASMCluster *cluster);
|
|
|
|
WASMDebugInstance *
|
|
wasm_exec_env_get_instance(WASMExecEnv *exec_env);
|
|
|
|
bool
|
|
wasm_debug_engine_init(char *ip_addr, int32 process_port);
|
|
|
|
void
|
|
wasm_debug_engine_destroy();
|
|
|
|
WASMExecEnv *
|
|
wasm_debug_instance_get_current_env(WASMDebugInstance *instance);
|
|
|
|
uint64
|
|
wasm_debug_instance_get_pid(WASMDebugInstance *instance);
|
|
|
|
korp_tid
|
|
wasm_debug_instance_get_tid(WASMDebugInstance *instance);
|
|
|
|
uint32
|
|
wasm_debug_instance_get_tids(WASMDebugInstance *instance, korp_tid tids[],
|
|
uint32 len);
|
|
|
|
void
|
|
wasm_debug_instance_set_cur_thread(WASMDebugInstance *instance, korp_tid tid);
|
|
|
|
uint64
|
|
wasm_debug_instance_get_pc(WASMDebugInstance *instance);
|
|
|
|
uint64
|
|
wasm_debug_instance_get_load_addr(WASMDebugInstance *instance);
|
|
|
|
WASMDebugMemoryInfo *
|
|
wasm_debug_instance_get_memregion(WASMDebugInstance *instance, uint64 addr);
|
|
|
|
void
|
|
wasm_debug_instance_destroy_memregion(WASMDebugInstance *instance,
|
|
WASMDebugMemoryInfo *mem_info);
|
|
|
|
bool
|
|
wasm_debug_instance_get_obj_mem(WASMDebugInstance *instance, uint64 addr,
|
|
char *buf, uint64 *size);
|
|
|
|
bool
|
|
wasm_debug_instance_get_linear_mem(WASMDebugInstance *instance, uint64 addr,
|
|
char *buf, uint64 *size);
|
|
|
|
bool
|
|
wasm_debug_instance_get_mem(WASMDebugInstance *instance, uint64 addr, char *buf,
|
|
uint64 *size);
|
|
|
|
bool
|
|
wasm_debug_instance_set_mem(WASMDebugInstance *instance, uint64 addr, char *buf,
|
|
uint64 *size);
|
|
|
|
uint32
|
|
wasm_debug_instance_get_call_stack_pcs(WASMDebugInstance *instance,
|
|
korp_tid tid, uint64 buf[], uint64 size);
|
|
|
|
bool
|
|
wasm_debug_instance_add_breakpoint(WASMDebugInstance *instance, uint64 addr,
|
|
uint64 length);
|
|
|
|
bool
|
|
wasm_debug_instance_remove_breakpoint(WASMDebugInstance *instance, uint64 addr,
|
|
uint64 length);
|
|
|
|
bool
|
|
wasm_debug_instance_watchpoint_write_add(WASMDebugInstance *instance,
|
|
uint64 addr, uint64 length);
|
|
|
|
bool
|
|
wasm_debug_instance_watchpoint_write_remove(WASMDebugInstance *instance,
|
|
uint64 addr, uint64 length);
|
|
|
|
bool
|
|
wasm_debug_instance_watchpoint_read_add(WASMDebugInstance *instance,
|
|
uint64 addr, uint64 length);
|
|
|
|
bool
|
|
wasm_debug_instance_watchpoint_read_remove(WASMDebugInstance *instance,
|
|
uint64 addr, uint64 length);
|
|
|
|
bool
|
|
wasm_debug_instance_on_failure(WASMDebugInstance *instance);
|
|
|
|
bool
|
|
wasm_debug_instance_interrupt_all_threads(WASMDebugInstance *instance);
|
|
|
|
bool
|
|
wasm_debug_instance_continue(WASMDebugInstance *instance);
|
|
|
|
bool
|
|
wasm_debug_instance_detach(WASMDebugInstance *instance);
|
|
|
|
bool
|
|
wasm_debug_instance_kill(WASMDebugInstance *instance);
|
|
|
|
uint32
|
|
wasm_debug_instance_get_thread_status(WASMDebugInstance *instance,
|
|
korp_tid tid);
|
|
|
|
bool
|
|
wasm_debug_instance_singlestep(WASMDebugInstance *instance, korp_tid tid);
|
|
|
|
bool
|
|
wasm_debug_instance_get_local(WASMDebugInstance *instance, int32 frame_index,
|
|
int32 local_index, char buf[], int32 *size);
|
|
|
|
bool
|
|
wasm_debug_instance_get_global(WASMDebugInstance *instance, int32 frame_index,
|
|
int32 global_index, char buf[], int32 *size);
|
|
|
|
#if WASM_ENABLE_LIBC_WASI != 0
|
|
bool
|
|
wasm_debug_instance_get_current_object_name(WASMDebugInstance *instance,
|
|
char name_buffer[], uint32 len);
|
|
#endif
|
|
|
|
uint64
|
|
wasm_debug_instance_mmap(WASMDebugInstance *instance, uint32 size,
|
|
int32 map_prot);
|
|
|
|
bool
|
|
wasm_debug_instance_ummap(WASMDebugInstance *instance, uint64 addr);
|
|
#endif
|